I use Storage
facades to manage my Laravel application files and folder a lot, either on server storage or S3 compatible storage like DigitalOcean Spaces.
Recently I have a new microservice project kicked start and using Laravel 9, and I use the Storage
facades as same as I did in previous projects.
So this time I need to use Storage::exists()
to check if a file exists in a huge size of DigitalOcean Spaces (around 300GB). But the speed is pretty slow, sometimes it takes 3 seconds, but sometimes it can take more than 10 seconds for a single file check.
This problem happened quite randomly because I use the same method to check in my old projects running with other Laravel versions doesn’t have such a problem. Even if I use another S3 client also couldn’t reproduce the slowness, thus I decided to dig in and see what was going on.
End up I found this in FilesystemAdapter.php
:
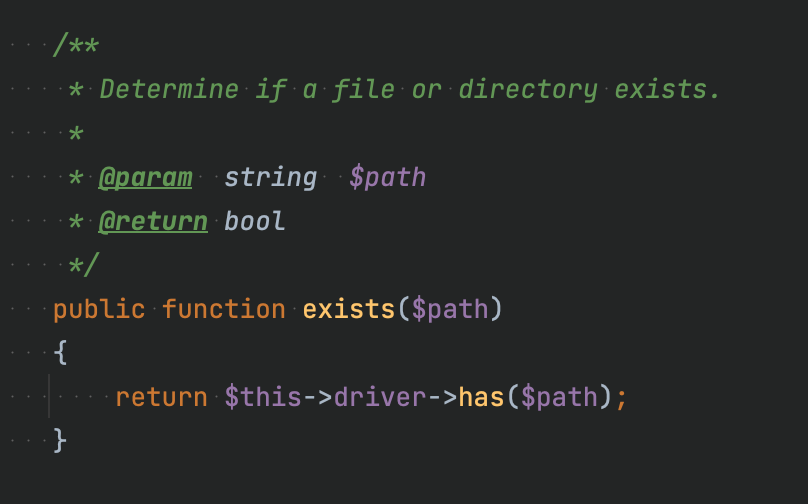
This leads me to the driver League\Flysystem\Filesystem
, and I can see the implementation of has()
function like the following:
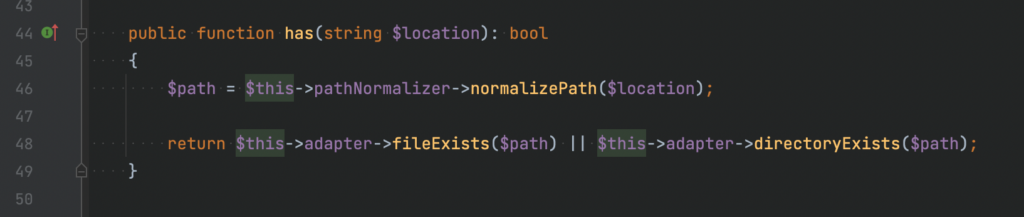
And YES. This explains why the checking of a single file takes longer than expected.
Workaround
Use Storage::disk('s3')->fileExists($path)
to check the file directly.
It avoids the calling to directoryExists($path)
, which probably causes slowness on a huge side S3 storage.